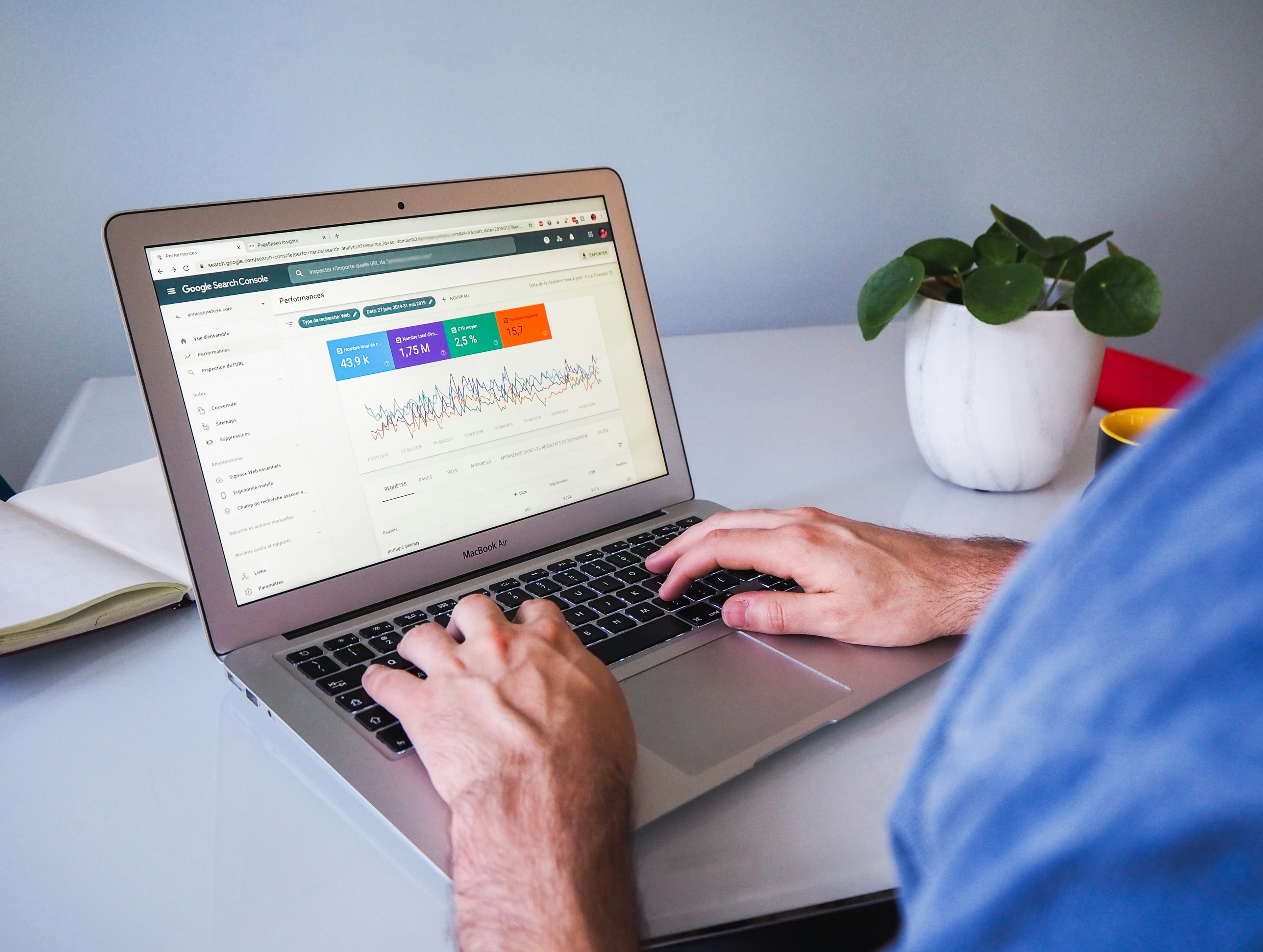
Running a Distributed Local LLM System: A Comprehensive Implementation Guide
The Power of Distributed Local LLMs: Breaking Hardware Limitations
The landscape of artificial intelligence has been transformed by large language models (LLMs), with options now extending beyond cloud-based APIs to running these powerful models locally. However, as models grow in size and complexity, the hardware requirements for running them effectively can become prohibitive for individual machines. This is where distributed systems come into play, allowing you to harness the combined resources of multiple computers to run larger, more capable models.
This comprehensive guide will walk you through the entire process of setting up a distributed local LLM system—from understanding the underlying concepts to advanced optimization techniques. Whether you're a researcher looking to experiment with larger models, an organization seeking to maintain data privacy while leveraging AI, or an enthusiast wanting to explore the frontiers of local AI deployment, this guide will provide you with the knowledge and tools to succeed.
Why Run LLMs in a Distributed Environment?
Before diving into technical details, it's worth understanding the advantages of distributed local LLM setups:
-
Running Larger Models: Distribute the memory and computational requirements of larger models across multiple machines, enabling the use of more powerful LLMs than any single computer could handle.
-
Improved Performance: Leverage the combined processing power of multiple GPUs across different machines to reduce inference time and increase throughput.
-
Resource Efficiency: Make use of existing hardware resources across your network rather than investing in expensive, specialized equipment.
-
Privacy and Control: Maintain complete control over your data and the inference process while still accessing powerful AI capabilities.
-
Fault Tolerance: Create systems that can continue operating even if individual nodes fail or become unavailable.
-
Scalability: Easily scale your system by adding more machines as your requirements grow.
Let's begin by exploring the foundational concepts of distributed LLM systems before moving to practical implementation.
Understanding Distributed LLM Architecture
A distributed LLM system splits the work of running a large language model across multiple computing nodes. There are several approaches to distributing this workload:
Tensor Parallelism
Tensor parallelism involves splitting individual tensors (the core mathematical structures in neural networks) across multiple devices. In the context of transformer-based LLMs, this often means dividing the attention heads or feed-forward layers across different GPUs or machines.
Key characteristics:
- Reduces per-device memory requirements
- Requires high-bandwidth, low-latency connections between devices
- Introduces some communication overhead during processing
- Typically implemented within specialized frameworks
Pipeline Parallelism
Pipeline parallelism divides the model sequentially, with different layers assigned to different devices. Each device processes its assigned layers and then passes the output to the next device in the pipeline.
Key characteristics:
- Works well for models with many sequential layers
- Reduces peak memory usage per device
- Can introduce latency as data moves through the pipeline
- May leave some devices idle at certain stages of processing
Model Parallelism
A more general approach that encompasses both tensor and pipeline parallelism, model parallelism refers to any technique that splits different parts of the model's architecture across multiple devices.
Data Parallelism
While less common for inference (and more relevant for training), data parallelism processes different input samples on different devices, each holding a complete copy of the model. This approach can be useful for high-throughput batch processing scenarios.
Hardware and Network Considerations
The effectiveness of your distributed LLM system depends significantly on your hardware configuration and network setup:
Hardware Requirements
Compute Nodes:
- Multiple machines with compatible GPUs (NVIDIA GPUs are currently most widely supported)
- Sufficient CPU and system RAM to support loading and preprocessing
- Fast storage (preferably SSD) for model weights and caching
Network Infrastructure:
- High-bandwidth, low-latency connections between nodes (10 Gigabit Ethernet minimum for serious implementations)
- Reliable network equipment capable of handling sustained high-throughput communication
- Minimal network hops between compute nodes
GPU Selection and Compatibility
Not all GPUs are created equal for distributed LLM workloads:
- NVIDIA A100, H100, or RTX 4090 GPUs offer excellent performance for LLM workloads
- Older generation cards (RTX 3090, 3080, etc.) can still be effective in a distributed setup
- Mixed GPU setups are possible but introduce additional complexity
Network Topology
The way you connect your compute nodes can significantly impact performance:
- Star topology: A central coordinator node connecting to worker nodes
- Mesh topology: All nodes connected to all other nodes
- Ring topology: Each node connected to two others in a circular arrangement
For most home or small lab setups, a simple star topology with a fast central switch often provides the best balance of performance and simplicity.
Setting Up the Software Environment
Now that we understand the architecture and hardware considerations, let's look at setting up the software environment for your distributed LLM system:
Base System Requirements
For each node in your cluster:
- A Linux-based operating system (Ubuntu 22.04 LTS is recommended for wide compatibility)
- NVIDIA drivers (if using NVIDIA GPUs)
- CUDA toolkit (version compatible with your chosen framework)
- Python 3.9+ with pip and venv or conda for environment management
Key Software Frameworks
Several frameworks support distributed LLM inference:
vLLM: A high-throughput and memory-efficient inference and serving engine with support for distributed inference.
pip install vllm
Text Generation Inference (TGI): Hugging Face's optimized framework for serving LLMs.
pip install text-generation-inference
LightLLM: A lightweight framework designed specifically for distributed LLM inference.
pip install lightllm
DeepSpeed Inference: Microsoft's framework with significant optimization for distributed scenarios.
pip install deepspeed
For this guide, we'll focus primarily on vLLM due to its strong performance and relative ease of setup, though the concepts apply across frameworks.
Implementing a Basic Distributed System with vLLM
Let's walk through the process of setting up a basic distributed LLM system using vLLM:
Step 1: Prepare Your Environment
On each node in your cluster, create a Python virtual environment and install the necessary packages:
# Create and activate a virtual environment
python -m venv vllm-env
source vllm-env/bin/activate
# Install vLLM and dependencies
pip install vllm
pip install ray # Ray is used for distributed computing
Step 2: Configure the Ray Cluster
Ray is the underlying distributed computing framework used by vLLM. You'll need to set up a Ray cluster with your machines:
- Start the Ray head node on your primary machine:
ray start --head --port=6379
This command will output an address that looks something like:
Ray cluster started. You can connect to this cluster by adding --address='192.168.1.100:6379' to your Ray command.
- Connect worker nodes to the head node by running the following on each worker machine:
ray start --address='192.168.1.100:6379' # Use the address from your head node
Step 3: Launch the Distributed LLM
Now you can start the distributed LLM using vLLM. On the head node:
import ray
from vllm.distributed import DistributedLLM
# Initialize the distributed LLM
distributed_llm = DistributedLLM(
model="meta-llama/Llama-2-70b-hf", # Specify the model to load
tensor_parallel_size=4, # Number of GPUs for tensor parallelism
dtype="bfloat16", # Use bfloat16 for efficiency
trust_remote_code=True
)
# Generate text with the distributed model
outputs = distributed_llm.generate(
["Explain how distributed computing works in simple terms"],
max_tokens=512,
temperature=0.7
)
# Print the generated text
for output in outputs:
print(output.text)
This basic example demonstrates how to set up a simple distributed inference system. The tensor_parallel_size
parameter determines how many GPUs will be used for tensor parallelism.
Advanced Configuration and Optimization
Once you have a basic system running, you can enhance its performance and capabilities through various optimizations:
Memory Optimization Techniques
Quantization: Reduce model precision to save memory:
distributed_llm = DistributedLLM(
model="meta-llama/Llama-2-70b-hf",
tensor_parallel_size=4,
dtype="int8", # Using 8-bit quantization
quantization="awq" # Specify quantization method
)
Continuous Batching: Process multiple requests simultaneously to improve throughput:
distributed_llm = DistributedLLM(
model="meta-llama/Llama-2-70b-hf",
tensor_parallel_size=4,
max_num_batched_tokens=4096, # Control batch size
max_num_seqs=10, # Maximum number of sequences to process
)
Paged Attention: vLLM's implementation of paged attention reduces memory fragmentation:
distributed_llm = DistributedLLM(
model="meta-llama/Llama-2-70b-hf",
tensor_parallel_size=4,
block_size=16, # Adjust block size for paged attention
)
Communication Optimization
NCCL Tuning: If using NVIDIA GPUs, tune the NCCL (NVIDIA Collective Communications Library) parameters for your specific network:
export NCCL_SOCKET_IFNAME=eth0 # Specify network interface
export NCCL_DEBUG=INFO # Enable debug info
export NCCL_IB_DISABLE=0 # Enable InfiniBand if available
export NCCL_P2P_DISABLE=0 # Enable P2P if available
Ray Tuning: Optimize Ray's distributed object store:
export RAY_memory_monitor_refresh_ms=0 # Disable memory monitoring
export RAY_object_store_memory=100000000000 # Set object store size
Load Balancing
Implementing a load balancing strategy helps distribute requests evenly across your cluster:
from vllm.distributed import DistributedLLM
from vllm.sampling import SamplingParams
import ray
# Start multiple model engines
@ray.remote(num_gpus=1)
class LLMWorker:
def __init__(self, model_name, tensor_parallel_size):
self.llm = DistributedLLM(
model=model_name,
tensor_parallel_size=tensor_parallel_size
)
def generate(self, prompt, params):
return self.llm.generate([prompt], SamplingParams(**params))[0].text
# Create workers
workers = [LLMWorker.remote("meta-llama/Llama-2-70b-hf", 4) for _ in range(num_workers)]
# Simple round-robin load balancer
def load_balance_request(prompt, params, current_idx=0):
worker = workers[current_idx % len(workers)]
current_idx += 1
return ray.get(worker.generate.remote(prompt, params))
Setting Up a REST API Server
To make your distributed LLM accessible to applications, you can create a REST API server:
Using FastAPI with vLLM
import ray
from fastapi import FastAPI, BackgroundTasks
from pydantic import BaseModel
from vllm.distributed import DistributedLLM
from vllm.sampling import SamplingParams
import uvicorn
import asyncio
app = FastAPI()
# Initialize Ray and the distributed LLM
ray.init(address="auto")
llm = DistributedLLM(
model="meta-llama/Llama-2-70b-hf",
tensor_parallel_size=4,
dtype="bfloat16"
)
class GenerationRequest(BaseModel):
prompt: str
max_tokens: int = 512
temperature: float = 0.7
top_p: float = 0.9
@app.post("/generate")
async def generate_text(request: GenerationRequest, background_tasks: BackgroundTasks):
# Create sampling parameters
sampling_params = SamplingParams(
max_tokens=request.max_tokens,
temperature=request.temperature,
top_p=request.top_p
)
# Run generation in a background task to avoid blocking
def generate():
outputs = llm.generate([request.prompt], sampling_params)
return outputs[0].text
# Run in a separate thread to avoid blocking the event loop
loop = asyncio.get_event_loop()
result = await loop.run_in_executor(None, generate)
return {"generated_text": result}
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8000)
Save this as api_server.py
and run it:
python api_server.py
Your API will be accessible at http://your_server_ip:8000/generate
.
Monitoring and Troubleshooting
A robust distributed system requires effective monitoring and troubleshooting capabilities:
Setting Up Monitoring
Ray Dashboard: Ray provides a built-in dashboard for monitoring your cluster:
# Start Ray with the dashboard
ray start --head --dashboard-host=0.0.0.0 --dashboard-port=8265
Access the dashboard at http://your_server_ip:8265
Prometheus and Grafana: For more comprehensive monitoring, set up Prometheus to collect metrics and Grafana to visualize them:
- Install Prometheus exporter in your application:
from prometheus_client import start_http_server, Counter, Gauge
# Metrics
request_count = Counter('llm_requests_total', 'Total number of requests')
request_latency = Gauge('llm_request_latency_seconds', 'Request latency in seconds')
gpu_memory_usage = Gauge('gpu_memory_usage_bytes', 'GPU memory usage in bytes', ['device'])
# Start Prometheus metrics server
start_http_server(8000)
# In your processing code
def process_request():
request_count.inc()
with request_latency.time():
# Your processing code here
- Configure Prometheus to scrape these metrics and Grafana to visualize them.
Common Issues and Solutions
GPU Out of Memory Errors:
- Reduce model precision (use int8 or int4 quantization)
- Increase tensor parallelism degree
- Reduce batch size or max sequence length
Network Communication Issues:
- Check network bandwidth between nodes with tools like
iperf
- Ensure all nodes can communicate with each other
- Verify NCCL settings and network interface configurations
Load Balancing Problems:
- Monitor request distribution across nodes
- Implement retry mechanisms for failed requests
- Consider adaptive load balancing based on node performance
Advanced Use Cases and Extensions
Once you have a basic distributed LLM system running, you can extend it for more specialized use cases:
Fine-tuning Support
Incorporate support for using your own fine-tuned models:
distributed_llm = DistributedLLM(
model="path/to/your/fine-tuned/model",
tensor_parallel_size=4,
dtype="bfloat16"
)
Retrieval-Augmented Generation (RAG)
Implement RAG to enhance your LLM with domain-specific knowledge:
from vllm.distributed import DistributedLLM
from langchain_community.vectorstores import FAISS
from langchain.embeddings import HuggingFaceEmbeddings
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.document_loaders import DirectoryLoader
# Set up document retrieval
loader = DirectoryLoader("path/to/documents/", glob="**/*.pdf")
documents = loader.load()
text_splitter = RecursiveCharacterTextSplitter(chunk_size=1000, chunk_overlap=100)
splits = text_splitter.split_documents(documents)
# Create vector store
embeddings = HuggingFaceEmbeddings(model_name="sentence-transformers/all-mpnet-base-v2")
vectorstore = FAISS.from_documents(splits, embeddings)
# Initialize the LLM
llm = DistributedLLM(
model="meta-llama/Llama-2-70b-hf",
tensor_parallel_size=4,
dtype="bfloat16"
)
# RAG function
def rag_generate(query, k=3):
# Retrieve relevant documents
relevant_docs = vectorstore.similarity_search(query, k=k)
context = "\n".join([doc.page_content for doc in relevant_docs])
# Construct the prompt with the context
prompt = f"""Context information is below.
---------------------
{context}
---------------------
Given the context information and not prior knowledge, answer the question: {query}
"""
# Generate the response
response = llm.generate([prompt], max_tokens=512)
return response[0].text
Multi-Model Deployment
Host multiple models on your distributed cluster:
# Initialize multiple models
llama_model = DistributedLLM(
model="meta-llama/Llama-2-70b-hf",
tensor_parallel_size=2, # Use fewer GPUs per model
dtype="bfloat16"
)
mistral_model = DistributedLLM(
model="mistralai/Mistral-7B-Instruct-v0.2",
tensor_parallel_size=1, # Smaller model needs fewer GPUs
dtype="bfloat16"
)
# Function to select the appropriate model based on the request
def route_request(prompt, model_name, params):
if model_name.lower() == "llama":
return llama_model.generate([prompt], params)[0].text
elif model_name.lower() == "mistral":
return mistral_model.generate([prompt], params)[0].text
else:
raise ValueError(f"Unknown model: {model_name}")
Scaling Beyond: Multi-Region and Hybrid Deployments
For organizations with global operations or specialized needs, more advanced distributed configurations may be appropriate:
Multi-Region Deployment
Deploy your distributed LLM system across multiple geographic regions for lower latency and higher availability:
- Set up regional Ray clusters in each geographic location
- Implement a global routing layer that directs requests to the nearest available cluster
- Establish synchronization mechanisms for sharing model updates and monitoring data
Hybrid Cloud-Edge Deployment
Combine local resources with cloud capabilities for flexible scaling:
- Deploy base clusters on-premises for regular workloads
- Configure cloud burst capability for handling peak loads
- Implement request routing logic that considers latency, cost, and privacy requirements
Conclusion: Building a Sustainable Distributed LLM Infrastructure
As you develop your distributed LLM system, keep these principles in mind for long-term success:
Sustainability Considerations
- Power Efficiency: Monitor and optimize power consumption, especially for 24/7 services
- Resource Scaling: Scale resources up and down based on actual demand
- Hardware Lifecycle: Plan for hardware refreshes and upgrades as technology evolves
Best Practices for Production Deployments
- Documentation: Maintain comprehensive documentation of your setup and configuration
- Automation: Automate deployment, scaling, and recovery procedures
- Security: Implement appropriate authentication, authorization, and data protection measures
- Regular Testing: Conduct load testing and failure scenario drills
The Future of Distributed LLMs
As LLM technology continues to evolve, distributed systems will likely become even more important, enabling:
- Even Larger Models: Running trillion-parameter models across commodity hardware
- Specialized Model Ensembles: Combining multiple specialized models for enhanced capabilities
- Edge-Optimized Architectures: New model designs specifically built for distributed edge deployment
- Privacy-Preserving Computation: Techniques like federated learning integrated with distributed inference
By mastering distributed LLM deployment today, you're preparing for an AI future where powerful models run anywhere and everywhere they're needed, with performance, privacy, and efficiency that cloud-only approaches cannot match.
Additional Resources
To deepen your understanding of distributed LLM systems, explore these resources:
- vLLM GitHub Repository: For the latest features and documentation
- Ray Documentation: For advanced distributed computing techniques
- Hugging Face Model Hub: For accessing and comparing different models
- NVIDIA Developer Forums: For GPU-specific optimization techniques
- MLOps Community: For best practices in deploying ML systems in production
With the approach outlined in this guide, you'll be well-equipped to build, optimize, and scale a distributed LLM system that meets your specific requirements while maintaining control over your data and infrastructure.